mirror of
https://github.com/hawkeye-stan/msfs-popout-panel-manager.git
synced 2024-10-16 14:10:45 +00:00
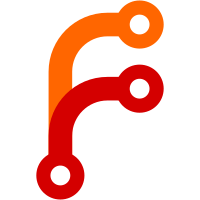
commit 2bbda3c4e4969fdf05566908fde01f1c9e4e23f7 Author: Stanley <hawkeyesk@outlook.com> Date: Mon Sep 5 23:54:39 2022 -0400 Added in-game panel support commit ec29b0ec2612b10e45ab9a73c10b88a98fcf6eaf Author: Stanley <hawkeyesk@outlook.com> Date: Sun Sep 4 21:21:37 2022 -0400 Added in-game panel support commit 97edc184f349e1fde74a15a643fb90fb9bd90395 Author: Stanley <hawkeyesk@outlook.com> Date: Thu Sep 1 18:08:44 2022 -0400 Update touch panel commit da48ca0a272290466952c5c1bd1ca035d56f930c Author: Stanley <hawkeyesk@outlook.com> Date: Mon Aug 29 22:19:38 2022 -0400 Added pop out panel temporary display commit 701346193804f93616b0e6e2222d9d55223f516f Author: Stanley <hawkeyesk@outlook.com> Date: Wed Aug 24 10:33:59 2022 -0400 Added auto resize window display mode commit 98cbcd949f1555b44db22267ce5c54858bef47cd Author: Stanley <hawkeyesk@outlook.com> Date: Wed Aug 24 09:39:38 2022 -0400 Added auto resize window display mode
176 lines
5.1 KiB
C#
176 lines
5.1 KiB
C#
using MSFSPopoutPanelManager.Shared;
|
|
using Newtonsoft.Json;
|
|
using PropertyChanged;
|
|
|
|
namespace MSFSPopoutPanelManager.UserDataAgent
|
|
{
|
|
public class AppSetting : ObservableObject
|
|
{
|
|
public AppSetting()
|
|
{
|
|
// Set defaults
|
|
LastUsedProfileId = -1;
|
|
AutoUpdaterUrl = "https://raw.githubusercontent.com/hawkeye-stan/msfs-popout-panel-manager/master/autoupdate.xml";
|
|
|
|
AlwaysOnTop = true;
|
|
MinimizeToTray = false;
|
|
StartMinimized = false;
|
|
|
|
AutoPopOutPanels = true;
|
|
|
|
UseAutoPanning = true;
|
|
AutoPanningKeyBinding = "0";
|
|
MinimizeAfterPopOut = false;
|
|
OnScreenMessageDuration = 1;
|
|
UseLeftRightControlToPopOut = false;
|
|
AfterPopOutCameraView = new AfterPopOutCameraView();
|
|
AfterPopOutCameraView.PropertyChanged += (source, e) =>
|
|
{
|
|
var arg = e as PropertyChangedExtendedEventArgs;
|
|
OnPropertyChanged(arg.PropertyName, arg.OldValue, arg.NewValue);
|
|
};
|
|
|
|
AutoDisableTrackIR = true;
|
|
|
|
AutoResizeMsfsGameWindow = true;
|
|
|
|
TouchScreenSettings = new TouchScreenSettings();
|
|
TouchScreenSettings.PropertyChanged += (source, e) =>
|
|
{
|
|
var arg = e as PropertyChangedExtendedEventArgs;
|
|
OnPropertyChanged(arg.PropertyName, arg.OldValue, arg.NewValue);
|
|
};
|
|
|
|
IsEnabledTouchPanelServer = false;
|
|
TouchPanelSettings = new TouchPanelSettings();
|
|
TouchPanelSettings.PropertyChanged += (source, e) =>
|
|
{
|
|
var arg = e as PropertyChangedExtendedEventArgs;
|
|
OnPropertyChanged(arg.PropertyName, arg.OldValue, arg.NewValue);
|
|
};
|
|
}
|
|
|
|
public string AutoUpdaterUrl { get; set; }
|
|
|
|
public int LastUsedProfileId { get; set; }
|
|
|
|
public bool MinimizeToTray { get; set; }
|
|
|
|
public bool AlwaysOnTop { get; set; }
|
|
|
|
public bool UseAutoPanning { get; set; }
|
|
|
|
public bool MinimizeAfterPopOut { get; set; }
|
|
|
|
public string AutoPanningKeyBinding { get; set; }
|
|
|
|
public bool StartMinimized { get; set; }
|
|
|
|
public bool AutoPopOutPanels { get; set; }
|
|
|
|
public bool AutoDisableTrackIR { get; set; }
|
|
|
|
public int OnScreenMessageDuration { get; set; }
|
|
|
|
public bool UseLeftRightControlToPopOut { get; set; }
|
|
|
|
public bool IsEnabledTouchPanelServer { get; set; }
|
|
|
|
public bool AutoResizeMsfsGameWindow { get; set; }
|
|
|
|
public AfterPopOutCameraView AfterPopOutCameraView { get; set; }
|
|
|
|
public TouchScreenSettings TouchScreenSettings { get; set; }
|
|
|
|
public TouchPanelSettings TouchPanelSettings { get; set; }
|
|
|
|
|
|
[JsonIgnore, DoNotNotify]
|
|
public bool AutoStart
|
|
{
|
|
get
|
|
{
|
|
return AppAutoStart.CheckIsAutoStart();
|
|
}
|
|
set
|
|
{
|
|
if (value)
|
|
AppAutoStart.Activate();
|
|
else
|
|
AppAutoStart.Deactivate();
|
|
}
|
|
}
|
|
}
|
|
|
|
public class AfterPopOutCameraView : ObservableObject
|
|
{
|
|
public AfterPopOutCameraView()
|
|
{
|
|
// Default values
|
|
EnableReturnToCameraView = true;
|
|
CameraView = AfterPopOutCameraViewType.CockpitCenterView;
|
|
CustomCameraKeyBinding = "1";
|
|
}
|
|
|
|
public bool EnableReturnToCameraView { get; set; }
|
|
|
|
public AfterPopOutCameraViewType CameraView { get; set; }
|
|
|
|
public string CustomCameraKeyBinding { get; set; }
|
|
|
|
// Use for MVVM binding only
|
|
[JsonIgnore]
|
|
public bool IsReturnToCustomCameraView { get { return CameraView == AfterPopOutCameraViewType.CustomCameraView; } }
|
|
|
|
// Use for MVVM binding only
|
|
[JsonIgnore]
|
|
public bool IsEnabledCustomCameraKeyBinding { get { return EnableReturnToCameraView && CameraView == AfterPopOutCameraViewType.CustomCameraView; } }
|
|
}
|
|
|
|
public enum AfterPopOutCameraViewType
|
|
{
|
|
CockpitCenterView,
|
|
CustomCameraView
|
|
}
|
|
|
|
public class TouchScreenSettings : ObservableObject
|
|
{
|
|
public TouchScreenSettings()
|
|
{
|
|
TouchDownUpDelay = 0;
|
|
RefocusGameWindow = true;
|
|
RefocusGameWindowDelay = 500;
|
|
}
|
|
|
|
public int TouchDownUpDelay { get; set; }
|
|
|
|
public bool RefocusGameWindow { get; set; }
|
|
|
|
public int RefocusGameWindowDelay { get; set; }
|
|
}
|
|
|
|
public class TouchPanelSettings : ObservableObject
|
|
{
|
|
public TouchPanelSettings()
|
|
{
|
|
// Default values
|
|
EnableTouchPanelIntegration = false;
|
|
DataRefreshInterval = 200;
|
|
MapRefreshInterval = 1000;
|
|
UseArduino = false;
|
|
EnableSound = true;
|
|
}
|
|
|
|
public bool EnableTouchPanelIntegration { get; set; }
|
|
|
|
public bool AutoStart { get; set; }
|
|
|
|
public int DataRefreshInterval { get; set; }
|
|
|
|
public int MapRefreshInterval { get; set; }
|
|
|
|
public bool UseArduino { get; set; }
|
|
|
|
public bool EnableSound { get; set; }
|
|
}
|
|
}
|