mirror of
https://github.com/immich-app/immich.git
synced 2025-01-10 13:56:47 +01:00
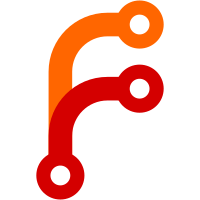
* add unicorn to eslint * fix lint errors for cli * fix merge * fix album name extraction * Update cli/src/commands/upload.command.ts Co-authored-by: Ben McCann <322311+benmccann@users.noreply.github.com> * es2k23 * use lowercase os * return undefined album name * fix bug in asset response dto * auto fix issues * fix server code style * es2022 and formatting * fix compilation error * fix test * fix config load * fix last lint errors * set string type * bump ts * start work on web * web formatting * Fix UUIDParamDto as UUIDParamDto * fix library service lint * fix web errors * fix errors * formatting * wip * lints fixed * web can now start * alphabetical package json * rename error * chore: clean up --------- Co-authored-by: Ben McCann <322311+benmccann@users.noreply.github.com> Co-authored-by: Jason Rasmussen <jrasm91@gmail.com>
51 lines
1.3 KiB
TypeScript
51 lines
1.3 KiB
TypeScript
import { derived, writable } from 'svelte/store';
|
|
|
|
export interface DownloadProgress {
|
|
progress: number;
|
|
total: number;
|
|
percentage: number;
|
|
abort: AbortController | null;
|
|
}
|
|
|
|
export const downloadAssets = writable<Record<string, DownloadProgress>>({});
|
|
|
|
export const isDownloading = derived(downloadAssets, ($downloadAssets) => {
|
|
if (Object.keys($downloadAssets).length === 0) {
|
|
return false;
|
|
}
|
|
|
|
return true;
|
|
});
|
|
|
|
const update = (key: string, value: Partial<DownloadProgress> | null) => {
|
|
downloadAssets.update((state) => {
|
|
const newState = { ...state };
|
|
|
|
if (value === null) {
|
|
delete newState[key];
|
|
return newState;
|
|
}
|
|
|
|
if (!newState[key]) {
|
|
newState[key] = { progress: 0, total: 0, percentage: 0, abort: null };
|
|
}
|
|
|
|
const item = newState[key];
|
|
Object.assign(item, value);
|
|
item.percentage = Math.min(Math.floor((item.progress / item.total) * 100), 100);
|
|
|
|
return newState;
|
|
});
|
|
};
|
|
|
|
export const downloadManager = {
|
|
add: (key: string, total: number, abort?: AbortController) => update(key, { total, abort }),
|
|
clear: (key: string) => update(key, null),
|
|
update: (key: string, progress: number, total?: number) => {
|
|
const download: Partial<DownloadProgress> = { progress };
|
|
if (total !== undefined) {
|
|
download.total = total;
|
|
}
|
|
update(key, download);
|
|
},
|
|
};
|