mirror of
https://github.com/immich-app/immich.git
synced 2025-03-29 03:09:38 +01:00
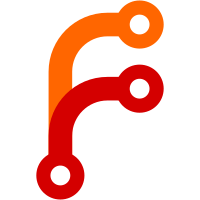
* fix(server): cannot render album page when all assets of an album are in trash * inner join * add e2e test * check empty albums too * render add to album button on empty album * lint * count 0 if undefined * fix album card test --------- Co-authored-by: mertalev <101130780+mertalev@users.noreply.github.com>
267 lines
9.5 KiB
TypeScript
267 lines
9.5 KiB
TypeScript
import { BadRequestException, Injectable } from '@nestjs/common';
|
|
import {
|
|
AddUsersDto,
|
|
AlbumInfoDto,
|
|
AlbumResponseDto,
|
|
AlbumStatisticsResponseDto,
|
|
CreateAlbumDto,
|
|
GetAlbumsDto,
|
|
UpdateAlbumDto,
|
|
mapAlbum,
|
|
mapAlbumWithAssets,
|
|
mapAlbumWithoutAssets,
|
|
} from 'src/dtos/album.dto';
|
|
import { BulkIdResponseDto, BulkIdsDto } from 'src/dtos/asset-ids.response.dto';
|
|
import { AuthDto } from 'src/dtos/auth.dto';
|
|
import { AlbumUserEntity } from 'src/entities/album-user.entity';
|
|
import { AlbumEntity } from 'src/entities/album.entity';
|
|
import { Permission } from 'src/enum';
|
|
import { AlbumAssetCount, AlbumInfoOptions } from 'src/interfaces/album.interface';
|
|
import { BaseService } from 'src/services/base.service';
|
|
import { addAssets, removeAssets } from 'src/utils/asset.util';
|
|
|
|
@Injectable()
|
|
export class AlbumService extends BaseService {
|
|
async getStatistics(auth: AuthDto): Promise<AlbumStatisticsResponseDto> {
|
|
const [owned, shared, notShared] = await Promise.all([
|
|
this.albumRepository.getOwned(auth.user.id),
|
|
this.albumRepository.getShared(auth.user.id),
|
|
this.albumRepository.getNotShared(auth.user.id),
|
|
]);
|
|
|
|
return {
|
|
owned: owned.length,
|
|
shared: shared.length,
|
|
notShared: notShared.length,
|
|
};
|
|
}
|
|
|
|
async getAll({ user: { id: ownerId } }: AuthDto, { assetId, shared }: GetAlbumsDto): Promise<AlbumResponseDto[]> {
|
|
await this.albumRepository.updateThumbnails();
|
|
|
|
let albums: AlbumEntity[];
|
|
if (assetId) {
|
|
albums = await this.albumRepository.getByAssetId(ownerId, assetId);
|
|
} else if (shared === true) {
|
|
albums = await this.albumRepository.getShared(ownerId);
|
|
} else if (shared === false) {
|
|
albums = await this.albumRepository.getNotShared(ownerId);
|
|
} else {
|
|
albums = await this.albumRepository.getOwned(ownerId);
|
|
}
|
|
|
|
// Get asset count for each album. Then map the result to an object:
|
|
// { [albumId]: assetCount }
|
|
const results = await this.albumRepository.getMetadataForIds(albums.map((album) => album.id));
|
|
const albumMetadata: Record<string, AlbumAssetCount> = {};
|
|
for (const metadata of results) {
|
|
albumMetadata[metadata.albumId] = metadata;
|
|
}
|
|
|
|
return Promise.all(
|
|
albums.map(async (album) => {
|
|
const lastModifiedAsset = await this.assetRepository.getLastUpdatedAssetForAlbumId(album.id);
|
|
return {
|
|
...mapAlbumWithoutAssets(album),
|
|
sharedLinks: undefined,
|
|
startDate: albumMetadata[album.id]?.startDate ?? undefined,
|
|
endDate: albumMetadata[album.id]?.endDate ?? undefined,
|
|
assetCount: albumMetadata[album.id]?.assetCount ?? 0,
|
|
lastModifiedAssetTimestamp: lastModifiedAsset?.updatedAt,
|
|
};
|
|
}),
|
|
);
|
|
}
|
|
|
|
async get(auth: AuthDto, id: string, dto: AlbumInfoDto): Promise<AlbumResponseDto> {
|
|
await this.requireAccess({ auth, permission: Permission.ALBUM_READ, ids: [id] });
|
|
await this.albumRepository.updateThumbnails();
|
|
const withAssets = dto.withoutAssets === undefined ? true : !dto.withoutAssets;
|
|
const album = await this.findOrFail(id, { withAssets });
|
|
const [albumMetadataForIds] = await this.albumRepository.getMetadataForIds([album.id]);
|
|
const lastModifiedAsset = await this.assetRepository.getLastUpdatedAssetForAlbumId(album.id);
|
|
|
|
return {
|
|
...mapAlbum(album, withAssets, auth),
|
|
startDate: albumMetadataForIds?.startDate ?? undefined,
|
|
endDate: albumMetadataForIds?.endDate ?? undefined,
|
|
assetCount: albumMetadataForIds?.assetCount ?? 0,
|
|
lastModifiedAssetTimestamp: lastModifiedAsset?.updatedAt,
|
|
};
|
|
}
|
|
|
|
async create(auth: AuthDto, dto: CreateAlbumDto): Promise<AlbumResponseDto> {
|
|
const albumUsers = dto.albumUsers || [];
|
|
|
|
for (const { userId } of albumUsers) {
|
|
const exists = await this.userRepository.get(userId, {});
|
|
if (!exists) {
|
|
throw new BadRequestException('User not found');
|
|
}
|
|
}
|
|
|
|
const allowedAssetIdsSet = await this.checkAccess({
|
|
auth,
|
|
permission: Permission.ASSET_SHARE,
|
|
ids: dto.assetIds || [],
|
|
});
|
|
const assetIds = [...allowedAssetIdsSet].map((id) => id);
|
|
|
|
const album = await this.albumRepository.create(
|
|
{
|
|
ownerId: auth.user.id,
|
|
albumName: dto.albumName,
|
|
description: dto.description,
|
|
albumThumbnailAssetId: assetIds[0] || null,
|
|
},
|
|
assetIds,
|
|
albumUsers,
|
|
);
|
|
|
|
for (const { userId } of albumUsers) {
|
|
await this.eventRepository.emit('album.invite', { id: album.id, userId });
|
|
}
|
|
|
|
return mapAlbumWithAssets(album);
|
|
}
|
|
|
|
async update(auth: AuthDto, id: string, dto: UpdateAlbumDto): Promise<AlbumResponseDto> {
|
|
await this.requireAccess({ auth, permission: Permission.ALBUM_UPDATE, ids: [id] });
|
|
|
|
const album = await this.findOrFail(id, { withAssets: true });
|
|
|
|
if (dto.albumThumbnailAssetId) {
|
|
const results = await this.albumRepository.getAssetIds(id, [dto.albumThumbnailAssetId]);
|
|
if (results.size === 0) {
|
|
throw new BadRequestException('Invalid album thumbnail');
|
|
}
|
|
}
|
|
const updatedAlbum = await this.albumRepository.update(album.id, {
|
|
id: album.id,
|
|
albumName: dto.albumName,
|
|
description: dto.description,
|
|
albumThumbnailAssetId: dto.albumThumbnailAssetId,
|
|
isActivityEnabled: dto.isActivityEnabled,
|
|
order: dto.order,
|
|
});
|
|
|
|
return mapAlbumWithoutAssets(updatedAlbum);
|
|
}
|
|
|
|
async delete(auth: AuthDto, id: string): Promise<void> {
|
|
await this.requireAccess({ auth, permission: Permission.ALBUM_DELETE, ids: [id] });
|
|
await this.albumRepository.delete(id);
|
|
}
|
|
|
|
async addAssets(auth: AuthDto, id: string, dto: BulkIdsDto): Promise<BulkIdResponseDto[]> {
|
|
const album = await this.findOrFail(id, { withAssets: false });
|
|
await this.requireAccess({ auth, permission: Permission.ALBUM_ADD_ASSET, ids: [id] });
|
|
|
|
const results = await addAssets(
|
|
auth,
|
|
{ access: this.accessRepository, bulk: this.albumRepository },
|
|
{ parentId: id, assetIds: dto.ids },
|
|
);
|
|
|
|
const { id: firstNewAssetId } = results.find(({ success }) => success) || {};
|
|
if (firstNewAssetId) {
|
|
await this.albumRepository.update(id, {
|
|
id,
|
|
updatedAt: new Date(),
|
|
albumThumbnailAssetId: album.albumThumbnailAssetId ?? firstNewAssetId,
|
|
});
|
|
|
|
const allUsersExceptUs = [...album.albumUsers.map(({ user }) => user.id), album.owner.id].filter(
|
|
(userId) => userId !== auth.user.id,
|
|
);
|
|
|
|
if (allUsersExceptUs.length > 0) {
|
|
await this.eventRepository.emit('album.update', { id, recipientIds: allUsersExceptUs });
|
|
}
|
|
}
|
|
|
|
return results;
|
|
}
|
|
|
|
async removeAssets(auth: AuthDto, id: string, dto: BulkIdsDto): Promise<BulkIdResponseDto[]> {
|
|
await this.requireAccess({ auth, permission: Permission.ALBUM_REMOVE_ASSET, ids: [id] });
|
|
|
|
const album = await this.findOrFail(id, { withAssets: false });
|
|
const results = await removeAssets(
|
|
auth,
|
|
{ access: this.accessRepository, bulk: this.albumRepository },
|
|
{ parentId: id, assetIds: dto.ids, canAlwaysRemove: Permission.ALBUM_DELETE },
|
|
);
|
|
|
|
const removedIds = results.filter(({ success }) => success).map(({ id }) => id);
|
|
if (removedIds.length > 0 && album.albumThumbnailAssetId && removedIds.includes(album.albumThumbnailAssetId)) {
|
|
await this.albumRepository.updateThumbnails();
|
|
}
|
|
|
|
return results;
|
|
}
|
|
|
|
async addUsers(auth: AuthDto, id: string, { albumUsers }: AddUsersDto): Promise<AlbumResponseDto> {
|
|
await this.requireAccess({ auth, permission: Permission.ALBUM_SHARE, ids: [id] });
|
|
|
|
const album = await this.findOrFail(id, { withAssets: false });
|
|
|
|
for (const { userId, role } of albumUsers) {
|
|
if (album.ownerId === userId) {
|
|
throw new BadRequestException('Cannot be shared with owner');
|
|
}
|
|
|
|
const exists = album.albumUsers.find(({ user: { id } }) => id === userId);
|
|
if (exists) {
|
|
throw new BadRequestException('User already added');
|
|
}
|
|
|
|
const user = await this.userRepository.get(userId, {});
|
|
if (!user) {
|
|
throw new BadRequestException('User not found');
|
|
}
|
|
|
|
await this.albumUserRepository.create({ usersId: userId, albumsId: id, role });
|
|
await this.eventRepository.emit('album.invite', { id, userId });
|
|
}
|
|
|
|
return this.findOrFail(id, { withAssets: true }).then(mapAlbumWithoutAssets);
|
|
}
|
|
|
|
async removeUser(auth: AuthDto, id: string, userId: string | 'me'): Promise<void> {
|
|
if (userId === 'me') {
|
|
userId = auth.user.id;
|
|
}
|
|
|
|
const album = await this.findOrFail(id, { withAssets: false });
|
|
|
|
if (album.ownerId === userId) {
|
|
throw new BadRequestException('Cannot remove album owner');
|
|
}
|
|
|
|
const exists = album.albumUsers.find(({ user: { id } }) => id === userId);
|
|
if (!exists) {
|
|
throw new BadRequestException('Album not shared with user');
|
|
}
|
|
|
|
// non-admin can remove themselves
|
|
if (auth.user.id !== userId) {
|
|
await this.requireAccess({ auth, permission: Permission.ALBUM_SHARE, ids: [id] });
|
|
}
|
|
|
|
await this.albumUserRepository.delete({ albumsId: id, usersId: userId });
|
|
}
|
|
|
|
async updateUser(auth: AuthDto, id: string, userId: string, dto: Partial<AlbumUserEntity>): Promise<void> {
|
|
await this.requireAccess({ auth, permission: Permission.ALBUM_SHARE, ids: [id] });
|
|
await this.albumUserRepository.update({ albumsId: id, usersId: userId }, { role: dto.role });
|
|
}
|
|
|
|
private async findOrFail(id: string, options: AlbumInfoOptions) {
|
|
const album = await this.albumRepository.getById(id, options);
|
|
if (!album) {
|
|
throw new BadRequestException('Album not found');
|
|
}
|
|
return album;
|
|
}
|
|
}
|