mirror of
https://github.com/immich-app/immich.git
synced 2025-01-04 02:46:47 +01:00
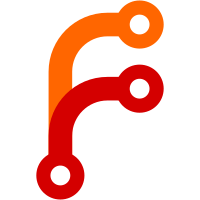
* feat: tags * fix: folder tree icons * navigate to tag from detail panel * delete tag * Tag position and add tag button * Tag asset in detail panel * refactor form * feat: navigate to tag page from clicking on a tag * feat: delete tags from the tag page * refactor: moving tag section in detail panel and add + tag button * feat: tag asset action in detail panel * refactor add tag form * fdisable add tag button when there is no selection * feat: tag bulk endpoint * feat: tag colors * chore: clean up * chore: unit tests * feat: write tags to sidecar * Remove tag and auto focus on tag creation form opened * chore: regenerate migration * chore: linting * add color picker to tag edit form * fix: force render tags timeline on navigating back from asset viewer * feat: read tags from keywords * chore: clean up --------- Co-authored-by: Alex Tran <alex.tran1502@gmail.com>
53 lines
1.8 KiB
TypeScript
53 lines
1.8 KiB
TypeScript
import { AlbumUserRole } from 'src/enum';
|
|
|
|
export const IAccessRepository = 'IAccessRepository';
|
|
|
|
export interface IAccessRepository {
|
|
activity: {
|
|
checkOwnerAccess(userId: string, activityIds: Set<string>): Promise<Set<string>>;
|
|
checkAlbumOwnerAccess(userId: string, activityIds: Set<string>): Promise<Set<string>>;
|
|
checkCreateAccess(userId: string, albumIds: Set<string>): Promise<Set<string>>;
|
|
};
|
|
|
|
asset: {
|
|
checkOwnerAccess(userId: string, assetIds: Set<string>): Promise<Set<string>>;
|
|
checkAlbumAccess(userId: string, assetIds: Set<string>): Promise<Set<string>>;
|
|
checkPartnerAccess(userId: string, assetIds: Set<string>): Promise<Set<string>>;
|
|
checkSharedLinkAccess(sharedLinkId: string, assetIds: Set<string>): Promise<Set<string>>;
|
|
};
|
|
|
|
authDevice: {
|
|
checkOwnerAccess(userId: string, deviceIds: Set<string>): Promise<Set<string>>;
|
|
};
|
|
|
|
album: {
|
|
checkOwnerAccess(userId: string, albumIds: Set<string>): Promise<Set<string>>;
|
|
checkSharedAlbumAccess(userId: string, albumIds: Set<string>, access: AlbumUserRole): Promise<Set<string>>;
|
|
checkSharedLinkAccess(sharedLinkId: string, albumIds: Set<string>): Promise<Set<string>>;
|
|
};
|
|
|
|
timeline: {
|
|
checkPartnerAccess(userId: string, partnerIds: Set<string>): Promise<Set<string>>;
|
|
};
|
|
|
|
memory: {
|
|
checkOwnerAccess(userId: string, memoryIds: Set<string>): Promise<Set<string>>;
|
|
};
|
|
|
|
person: {
|
|
checkFaceOwnerAccess(userId: string, assetFaceId: Set<string>): Promise<Set<string>>;
|
|
checkOwnerAccess(userId: string, personIds: Set<string>): Promise<Set<string>>;
|
|
};
|
|
|
|
partner: {
|
|
checkUpdateAccess(userId: string, partnerIds: Set<string>): Promise<Set<string>>;
|
|
};
|
|
|
|
stack: {
|
|
checkOwnerAccess(userId: string, stackIds: Set<string>): Promise<Set<string>>;
|
|
};
|
|
|
|
tag: {
|
|
checkOwnerAccess(userId: string, tagIds: Set<string>): Promise<Set<string>>;
|
|
};
|
|
}
|