mirror of
https://github.com/immich-app/immich.git
synced 2025-01-10 13:56:47 +01:00
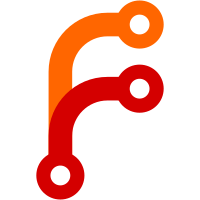
* add unicorn to eslint * fix lint errors for cli * fix merge * fix album name extraction * Update cli/src/commands/upload.command.ts Co-authored-by: Ben McCann <322311+benmccann@users.noreply.github.com> * es2k23 * use lowercase os * return undefined album name * fix bug in asset response dto * auto fix issues * fix server code style * es2022 and formatting * fix compilation error * fix test * fix config load * fix last lint errors * set string type * bump ts * start work on web * web formatting * Fix UUIDParamDto as UUIDParamDto * fix library service lint * fix web errors * fix errors * formatting * wip * lints fixed * web can now start * alphabetical package json * rename error * chore: clean up --------- Co-authored-by: Ben McCann <322311+benmccann@users.noreply.github.com> Co-authored-by: Jason Rasmussen <jrasm91@gmail.com>
32 lines
1,013 B
TypeScript
32 lines
1,013 B
TypeScript
import { ICryptoRepository } from '@app/domain';
|
|
import { Injectable } from '@nestjs/common';
|
|
import { compareSync, hash } from 'bcrypt';
|
|
import { createHash, randomBytes, randomUUID } from 'node:crypto';
|
|
import { createReadStream } from 'node:fs';
|
|
|
|
@Injectable()
|
|
export class CryptoRepository implements ICryptoRepository {
|
|
randomUUID = randomUUID;
|
|
randomBytes = randomBytes;
|
|
|
|
hashBcrypt = hash;
|
|
compareBcrypt = compareSync;
|
|
|
|
hashSha256(value: string) {
|
|
return createHash('sha256').update(value).digest('base64');
|
|
}
|
|
|
|
hashSha1(value: string | Buffer): Buffer {
|
|
return createHash('sha1').update(value).digest();
|
|
}
|
|
|
|
hashFile(filepath: string | Buffer): Promise<Buffer> {
|
|
return new Promise<Buffer>((resolve, reject) => {
|
|
const hash = createHash('sha1');
|
|
const stream = createReadStream(filepath);
|
|
stream.on('error', (error) => reject(error));
|
|
stream.on('data', (chunk) => hash.update(chunk));
|
|
stream.on('end', () => resolve(hash.digest()));
|
|
});
|
|
}
|
|
}
|