mirror of
https://github.com/immich-app/immich.git
synced 2025-01-10 13:56:47 +01:00
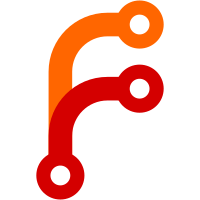
* feat: auto-stack burst photos * feat: move stacks to asset stack entity * chore: pin node version with volta in server * chore: update e2e cases * chore: cleanup * feat: migrate existing stacks --------- Co-authored-by: Jason Rasmussen <jrasm91@gmail.com>
47 lines
1.2 KiB
TypeScript
47 lines
1.2 KiB
TypeScript
import { IAssetStackRepository } from '@app/domain';
|
|
import { Injectable } from '@nestjs/common';
|
|
import { InjectRepository } from '@nestjs/typeorm';
|
|
import { Repository } from 'typeorm';
|
|
import { AssetStackEntity } from '../entities';
|
|
|
|
@Injectable()
|
|
export class AssetStackRepository implements IAssetStackRepository {
|
|
constructor(@InjectRepository(AssetStackEntity) private repository: Repository<AssetStackEntity>) {}
|
|
|
|
create(entity: Partial<AssetStackEntity>) {
|
|
return this.save(entity);
|
|
}
|
|
|
|
async delete(id: string): Promise<void> {
|
|
await this.repository.delete(id);
|
|
}
|
|
|
|
update(entity: Partial<AssetStackEntity>) {
|
|
return this.save(entity);
|
|
}
|
|
|
|
async getById(id: string): Promise<AssetStackEntity | null> {
|
|
return this.repository.findOne({
|
|
where: {
|
|
id,
|
|
},
|
|
relations: {
|
|
primaryAsset: true,
|
|
assets: true,
|
|
},
|
|
});
|
|
}
|
|
|
|
private async save(entity: Partial<AssetStackEntity>) {
|
|
const { id } = await this.repository.save(entity);
|
|
return this.repository.findOneOrFail({
|
|
where: {
|
|
id,
|
|
},
|
|
relations: {
|
|
primaryAsset: true,
|
|
assets: true,
|
|
},
|
|
});
|
|
}
|
|
}
|