mirror of
https://github.com/immich-app/immich.git
synced 2025-01-09 21:36:46 +01:00
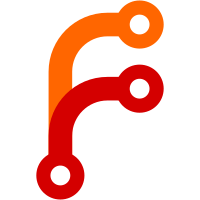
* feat: add admin config module for user configured config, uses it for ffmpeg * feat: add api endpoint to retrieve admin config settings and values * feat: add settings panel to admin page on web (wip) * feat: add api endpoint to update the admin config * chore: re-generate openapi spec after rebase * refactor: move from admin config to system config naming * chore: move away from UseGuards to new @Authenticated decorator * style: dark mode styling for lists and fix conflicting colors * wip: 2 column design, no edit button * refactor: system config * chore: generate open api * chore: rm broken test * chore: cleanup types * refactor: config module names Co-authored-by: Zack Pollard <zackpollard@ymail.com> Co-authored-by: Zack Pollard <zack.pollard@moonpig.com>
104 lines
3.4 KiB
TypeScript
104 lines
3.4 KiB
TypeScript
import { immichAppConfig } from '@app/common/config';
|
|
import { DatabaseModule } from '@app/database';
|
|
import { AssetEntity } from '@app/database/entities/asset.entity';
|
|
import { ExifEntity } from '@app/database/entities/exif.entity';
|
|
import { SmartInfoEntity } from '@app/database/entities/smart-info.entity';
|
|
import { UserEntity } from '@app/database/entities/user.entity';
|
|
import { QueueNameEnum } from '@app/job/constants/queue-name.constant';
|
|
import { BullModule } from '@nestjs/bull';
|
|
import { Module } from '@nestjs/common';
|
|
import { ConfigModule } from '@nestjs/config';
|
|
import { TypeOrmModule } from '@nestjs/typeorm';
|
|
import { ImmichConfigModule } from 'libs/immich-config/src';
|
|
import { CommunicationModule } from '../../immich/src/api-v1/communication/communication.module';
|
|
import { MicroservicesService } from './microservices.service';
|
|
import { AssetUploadedProcessor } from './processors/asset-uploaded.processor';
|
|
import { GenerateChecksumProcessor } from './processors/generate-checksum.processor';
|
|
import { MachineLearningProcessor } from './processors/machine-learning.processor';
|
|
import { MetadataExtractionProcessor } from './processors/metadata-extraction.processor';
|
|
import { ThumbnailGeneratorProcessor } from './processors/thumbnail.processor';
|
|
import { VideoTranscodeProcessor } from './processors/video-transcode.processor';
|
|
|
|
@Module({
|
|
imports: [
|
|
ConfigModule.forRoot(immichAppConfig),
|
|
DatabaseModule,
|
|
ImmichConfigModule,
|
|
TypeOrmModule.forFeature([UserEntity, ExifEntity, AssetEntity, SmartInfoEntity]),
|
|
BullModule.forRootAsync({
|
|
useFactory: async () => ({
|
|
prefix: 'immich_bull',
|
|
redis: {
|
|
host: process.env.REDIS_HOSTNAME || 'immich_redis',
|
|
port: parseInt(process.env.REDIS_PORT || '6379'),
|
|
db: parseInt(process.env.REDIS_DBINDEX || '0'),
|
|
password: process.env.REDIS_PASSWORD || undefined,
|
|
path: process.env.REDIS_SOCKET || undefined,
|
|
},
|
|
}),
|
|
}),
|
|
BullModule.registerQueue(
|
|
{
|
|
name: QueueNameEnum.THUMBNAIL_GENERATION,
|
|
defaultJobOptions: {
|
|
attempts: 3,
|
|
removeOnComplete: true,
|
|
removeOnFail: false,
|
|
},
|
|
},
|
|
{
|
|
name: QueueNameEnum.ASSET_UPLOADED,
|
|
defaultJobOptions: {
|
|
attempts: 3,
|
|
removeOnComplete: true,
|
|
removeOnFail: false,
|
|
},
|
|
},
|
|
{
|
|
name: QueueNameEnum.METADATA_EXTRACTION,
|
|
defaultJobOptions: {
|
|
attempts: 3,
|
|
removeOnComplete: true,
|
|
removeOnFail: false,
|
|
},
|
|
},
|
|
{
|
|
name: QueueNameEnum.VIDEO_CONVERSION,
|
|
defaultJobOptions: {
|
|
attempts: 3,
|
|
removeOnComplete: true,
|
|
removeOnFail: false,
|
|
},
|
|
},
|
|
{
|
|
name: QueueNameEnum.CHECKSUM_GENERATION,
|
|
defaultJobOptions: {
|
|
attempts: 3,
|
|
removeOnComplete: true,
|
|
removeOnFail: false,
|
|
},
|
|
},
|
|
{
|
|
name: QueueNameEnum.MACHINE_LEARNING,
|
|
defaultJobOptions: {
|
|
attempts: 3,
|
|
removeOnComplete: true,
|
|
removeOnFail: false,
|
|
},
|
|
},
|
|
),
|
|
CommunicationModule,
|
|
],
|
|
controllers: [],
|
|
providers: [
|
|
MicroservicesService,
|
|
AssetUploadedProcessor,
|
|
ThumbnailGeneratorProcessor,
|
|
MetadataExtractionProcessor,
|
|
VideoTranscodeProcessor,
|
|
GenerateChecksumProcessor,
|
|
MachineLearningProcessor,
|
|
],
|
|
exports: [],
|
|
})
|
|
export class MicroservicesModule {}
|