mirror of
https://github.com/immich-app/immich.git
synced 2025-01-09 13:26:47 +01:00
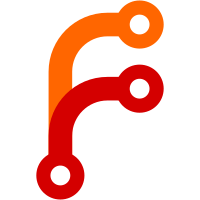
* fix(server): Split database queries based on PostgreSQL bound params limit PostgreSQL uses a 16-bit integer to indicate the number of bound parameters. This means that the maximum number of parameters for any query is 65535. Any query that tries to bind more than that (e.g. searching by a list of IDs) requires splitting the query into multiple chunks. This change includes refactoring every Repository that runs queries using a list of ids, and either flattening or merging results. Fixes #5788, #5997. Also, potentially a fix for #4648 (at least based on [this comment](https://github.com/immich-app/immich/issues/4648#issuecomment-1826134027)). References: * https://github.com/typeorm/typeorm/issues/7565 * [PostgreSQL message format - Bind](https://www.postgresql.org/docs/15/protocol-message-formats.html#PROTOCOL-MESSAGE-FORMATS-BIND) * misc: Create Chunked decorator to simplify implementation * feat: Add ChunkedArray/ChunkedSet decorators
27 lines
1 KiB
TypeScript
27 lines
1 KiB
TypeScript
import { SetMetadata } from '@nestjs/common';
|
|
|
|
export const GENERATE_SQL_KEY = 'generate-sql-key';
|
|
|
|
export interface GenerateSqlQueries {
|
|
name?: string;
|
|
params?: any[];
|
|
}
|
|
|
|
/** Decorator to enable versioning/tracking of generated Sql */
|
|
export const GenerateSql = (...options: GenerateSqlQueries[]) => SetMetadata(GENERATE_SQL_KEY, options);
|
|
|
|
export const DummyValue = {
|
|
UUID: '00000000-0000-4000-a000-000000000000',
|
|
PAGINATION: { take: 10, skip: 0 },
|
|
EMAIL: 'user@immich.app',
|
|
STRING: 'abcdefghi',
|
|
BUFFER: Buffer.from('abcdefghi'),
|
|
DATE: new Date(),
|
|
TIME_BUCKET: '2024-01-01T00:00:00.000Z',
|
|
};
|
|
|
|
// PostgreSQL uses a 16-bit integer to indicate the number of bound parameters. This means that the
|
|
// maximum number of parameters is 65535. Any query that tries to bind more than that (e.g. searching
|
|
// by a list of IDs) requires splitting the query into multiple chunks.
|
|
// We are rounding down this limit, as queries commonly include other filters and parameters.
|
|
export const DATABASE_PARAMETER_CHUNK_SIZE = 65500;
|