mirror of
https://github.com/immich-app/immich.git
synced 2025-03-01 15:11:21 +01:00
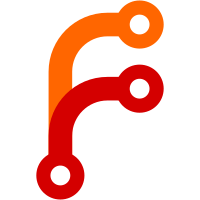
* feat(server): audit log * feedback * Insert to database * migration * test * controller/repository/service * test * module * feat(server): implement audit endpoint * directly return changed assets * add daily cleanup of audit table * fix tests * review feedback * ci * refactor(server): audit implementation * chore: open api --------- Co-authored-by: Alex Tran <alex.tran1502@gmail.com> Co-authored-by: Fynn Petersen-Frey <zoodyy@users.noreply.github.com> Co-authored-by: Jason Rasmussen <jrasm91@gmail.com>
26 lines
950 B
TypeScript
26 lines
950 B
TypeScript
import { AuditSearch, IAuditRepository } from '@app/domain';
|
|
import { InjectRepository } from '@nestjs/typeorm';
|
|
import { LessThan, MoreThan, Repository } from 'typeorm';
|
|
import { AuditEntity } from '../entities';
|
|
|
|
export class AuditRepository implements IAuditRepository {
|
|
constructor(@InjectRepository(AuditEntity) private repository: Repository<AuditEntity>) {}
|
|
|
|
getAfter(since: Date, options: AuditSearch): Promise<AuditEntity[]> {
|
|
return this.repository
|
|
.createQueryBuilder('audit')
|
|
.where({
|
|
createdAt: MoreThan(since),
|
|
action: options.action,
|
|
entityType: options.entityType,
|
|
ownerId: options.ownerId,
|
|
})
|
|
.distinctOn(['audit.entityId', 'audit.entityType'])
|
|
.orderBy('audit.entityId, audit.entityType, audit.createdAt', 'DESC')
|
|
.getMany();
|
|
}
|
|
|
|
async removeBefore(before: Date): Promise<void> {
|
|
await this.repository.delete({ createdAt: LessThan(before) });
|
|
}
|
|
}
|