mirror of
https://github.com/immich-app/immich.git
synced 2025-01-09 13:26:47 +01:00
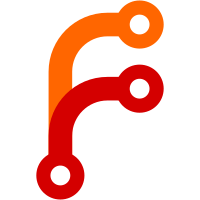
* feat(server): pagination for asset queries in jobs * default mock value for getAll * remove live photo name correction * order paginated results by createdAt * change log level * move usePagination to domain
59 lines
1.5 KiB
TypeScript
59 lines
1.5 KiB
TypeScript
import { basename, extname } from 'node:path';
|
|
|
|
export function getFileNameWithoutExtension(path: string): string {
|
|
return basename(path, extname(path));
|
|
}
|
|
|
|
export function getLivePhotoMotionFilename(stillName: string, motionName: string) {
|
|
return getFileNameWithoutExtension(stillName) + extname(motionName);
|
|
}
|
|
|
|
const KiB = Math.pow(1024, 1);
|
|
const MiB = Math.pow(1024, 2);
|
|
const GiB = Math.pow(1024, 3);
|
|
const TiB = Math.pow(1024, 4);
|
|
const PiB = Math.pow(1024, 5);
|
|
|
|
export const HumanReadableSize = { KiB, MiB, GiB, TiB, PiB };
|
|
|
|
export function asHumanReadable(bytes: number, precision = 1): string {
|
|
const units = ['B', 'KiB', 'MiB', 'GiB', 'TiB', 'PiB', 'EiB'];
|
|
|
|
let magnitude = 0;
|
|
let remainder = bytes;
|
|
while (remainder >= 1024) {
|
|
if (magnitude + 1 < units.length) {
|
|
magnitude++;
|
|
remainder /= 1024;
|
|
} else {
|
|
break;
|
|
}
|
|
}
|
|
|
|
return `${remainder.toFixed(magnitude == 0 ? 0 : precision)} ${units[magnitude]}`;
|
|
}
|
|
|
|
export interface PaginationOptions {
|
|
take: number;
|
|
skip?: number;
|
|
}
|
|
|
|
export interface PaginationResult<T> {
|
|
items: T[];
|
|
hasNextPage: boolean;
|
|
}
|
|
|
|
export type Paginated<T> = Promise<PaginationResult<T>>;
|
|
|
|
export async function* usePagination<T>(
|
|
pageSize: number,
|
|
getNextPage: (pagination: PaginationOptions) => Paginated<T>,
|
|
) {
|
|
let hasNextPage = true;
|
|
|
|
for (let skip = 0; hasNextPage; skip += pageSize) {
|
|
const result = await getNextPage({ take: pageSize, skip });
|
|
hasNextPage = result.hasNextPage;
|
|
yield result.items;
|
|
}
|
|
}
|