mirror of
https://github.com/immich-app/immich.git
synced 2025-01-07 20:36:48 +01:00
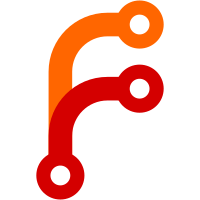
* Add Exif-Rating * Integrate star rating as own component * Add e2e tests for rating and validation * Rename component and async handleChangeRating * Display rating can be enabled in app settings * Correct i18n reference Co-authored-by: Michel Heusschen <59014050+michelheusschen@users.noreply.github.com> * Star rating: change from slider to buttons * Star rating for clarity * Design updates. * Renaming and code optimization * chore: clean up * chore: e2e formatting * light mode border and default value --------- Co-authored-by: Christoph Suter <christoph@suter-burri.ch> Co-authored-by: Michel Heusschen <59014050+michelheusschen@users.noreply.github.com> Co-authored-by: Mert <101130780+mertalev@users.noreply.github.com> Co-authored-by: Jason Rasmussen <jrasm91@gmail.com> Co-authored-by: Alex Tran <alex.tran1502@gmail.com>
104 lines
2.7 KiB
TypeScript
104 lines
2.7 KiB
TypeScript
import { AssetEntity } from 'src/entities/asset.entity';
|
|
import { Index, JoinColumn, OneToOne, PrimaryColumn } from 'typeorm';
|
|
import { Column } from 'typeorm/decorator/columns/Column.js';
|
|
import { Entity } from 'typeorm/decorator/entity/Entity.js';
|
|
|
|
@Entity('exif')
|
|
export class ExifEntity {
|
|
@OneToOne(() => AssetEntity, { onDelete: 'CASCADE', nullable: true })
|
|
@JoinColumn()
|
|
asset?: AssetEntity;
|
|
|
|
@PrimaryColumn()
|
|
assetId!: string;
|
|
|
|
/* General info */
|
|
@Column({ type: 'text', default: '' })
|
|
description!: string; // or caption
|
|
|
|
@Column({ type: 'integer', nullable: true })
|
|
exifImageWidth!: number | null;
|
|
|
|
@Column({ type: 'integer', nullable: true })
|
|
exifImageHeight!: number | null;
|
|
|
|
@Column({ type: 'bigint', nullable: true })
|
|
fileSizeInByte!: number | null;
|
|
|
|
@Column({ type: 'varchar', nullable: true })
|
|
orientation!: string | null;
|
|
|
|
@Column({ type: 'timestamptz', nullable: true })
|
|
dateTimeOriginal!: Date | null;
|
|
|
|
@Column({ type: 'timestamptz', nullable: true })
|
|
modifyDate!: Date | null;
|
|
|
|
@Column({ type: 'varchar', nullable: true })
|
|
timeZone!: string | null;
|
|
|
|
@Column({ type: 'float', nullable: true })
|
|
latitude!: number | null;
|
|
|
|
@Column({ type: 'float', nullable: true })
|
|
longitude!: number | null;
|
|
|
|
@Column({ type: 'varchar', nullable: true })
|
|
projectionType!: string | null;
|
|
|
|
@Index('exif_city')
|
|
@Column({ type: 'varchar', nullable: true })
|
|
city!: string | null;
|
|
|
|
@Index('IDX_live_photo_cid')
|
|
@Column({ type: 'varchar', nullable: true })
|
|
livePhotoCID!: string | null;
|
|
|
|
@Index('IDX_auto_stack_id')
|
|
@Column({ type: 'varchar', nullable: true })
|
|
autoStackId!: string | null;
|
|
|
|
@Column({ type: 'varchar', nullable: true })
|
|
state!: string | null;
|
|
|
|
@Column({ type: 'varchar', nullable: true })
|
|
country!: string | null;
|
|
|
|
/* Image info */
|
|
@Column({ type: 'varchar', nullable: true })
|
|
make!: string | null;
|
|
|
|
@Column({ type: 'varchar', nullable: true })
|
|
model!: string | null;
|
|
|
|
@Column({ type: 'varchar', nullable: true })
|
|
lensModel!: string | null;
|
|
|
|
@Column({ type: 'float8', nullable: true })
|
|
fNumber!: number | null;
|
|
|
|
@Column({ type: 'float8', nullable: true })
|
|
focalLength!: number | null;
|
|
|
|
@Column({ type: 'integer', nullable: true })
|
|
iso!: number | null;
|
|
|
|
@Column({ type: 'varchar', nullable: true })
|
|
exposureTime!: string | null;
|
|
|
|
@Column({ type: 'varchar', nullable: true })
|
|
profileDescription!: string | null;
|
|
|
|
@Column({ type: 'varchar', nullable: true })
|
|
colorspace!: string | null;
|
|
|
|
@Column({ type: 'integer', nullable: true })
|
|
bitsPerSample!: number | null;
|
|
|
|
@Column({ type: 'integer', nullable: true })
|
|
rating!: number | null;
|
|
|
|
/* Video info */
|
|
@Column({ type: 'float8', nullable: true })
|
|
fps?: number | null;
|
|
}
|