mirror of
https://github.com/immich-app/immich.git
synced 2025-01-09 13:26:47 +01:00
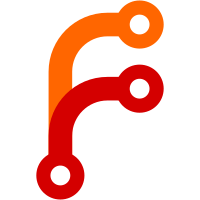
* feat: admin repair orphans tool * chore: open api * fix: include upload folder * fix: bugs * feat: empty placeholder * fix: checks * feat: move buttons to top of page * feat: styling and clipboard * styling * better clicking hitbox * fix: show title on hover * feat: download report * restrict file access to immich related files * Add description --------- Co-authored-by: Alex Tran <alex.tran1502@gmail.com> Co-authored-by: Daniel Dietzler <mail@ddietzler.dev>
74 lines
1.7 KiB
TypeScript
74 lines
1.7 KiB
TypeScript
import { AssetPathType, EntityType, PathType, PersonPathType, UserPathType } from '@app/infra/entities';
|
|
import { ApiProperty } from '@nestjs/swagger';
|
|
import { Type } from 'class-transformer';
|
|
import { IsArray, IsDate, IsEnum, IsString, IsUUID, ValidateNested } from 'class-validator';
|
|
import { Optional, ValidateUUID } from '../domain.util';
|
|
|
|
const PathEnum = Object.values({ ...AssetPathType, ...PersonPathType, ...UserPathType });
|
|
|
|
export class AuditDeletesDto {
|
|
@IsDate()
|
|
@Type(() => Date)
|
|
after!: Date;
|
|
|
|
@ApiProperty({ enum: EntityType, enumName: 'EntityType' })
|
|
@IsEnum(EntityType)
|
|
entityType!: EntityType;
|
|
|
|
@Optional()
|
|
@IsUUID('4')
|
|
@ApiProperty({ format: 'uuid' })
|
|
userId?: string;
|
|
}
|
|
|
|
export enum PathEntityType {
|
|
ASSET = 'asset',
|
|
PERSON = 'person',
|
|
USER = 'user',
|
|
}
|
|
|
|
export class AuditDeletesResponseDto {
|
|
needsFullSync!: boolean;
|
|
ids!: string[];
|
|
}
|
|
|
|
export class FileReportDto {
|
|
orphans!: FileReportItemDto[];
|
|
extras!: string[];
|
|
}
|
|
|
|
export class FileChecksumDto {
|
|
@IsString({ each: true })
|
|
filenames!: string[];
|
|
}
|
|
|
|
export class FileChecksumResponseDto {
|
|
filename!: string;
|
|
checksum!: string;
|
|
}
|
|
|
|
export class FileReportFixDto {
|
|
@IsArray()
|
|
@ValidateNested({ each: true })
|
|
@Type(() => FileReportItemDto)
|
|
items!: FileReportItemDto[];
|
|
}
|
|
|
|
// used both as request and response dto
|
|
export class FileReportItemDto {
|
|
@ValidateUUID()
|
|
entityId!: string;
|
|
|
|
@ApiProperty({ enumName: 'PathEntityType', enum: PathEntityType })
|
|
@IsEnum(PathEntityType)
|
|
entityType!: PathEntityType;
|
|
|
|
@ApiProperty({ enumName: 'PathType', enum: PathEnum })
|
|
@IsEnum(PathEnum)
|
|
pathType!: PathType;
|
|
|
|
@IsString()
|
|
pathValue!: string;
|
|
|
|
checksum?: string;
|
|
}
|