mirror of
https://github.com/immich-app/immich.git
synced 2025-01-10 13:56:47 +01:00
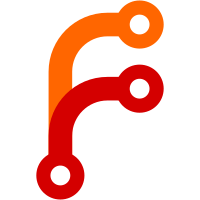
* feat(web): shuffle slideshow order * Fix play/stop issues * Enter/exit fullscreen mode * Prevent navigation to the next asset after exiting slideshow mode * Fix entering the slideshow mode from an album page * Simplify markup of the AssetViewer Group viewer area and navigation (prev/next/slideshow bar) controls together * Select a random asset from a random bucket * Preserve assets order in random mode * Exit fullscreen mode only if it is active * Extract SlideshowHistory class * Use traditional functions instead of arrow functions * Refactor SlideshowHistory class * Extract SlideshowBar component * Fix comments * Hide Say something in slideshow mode --------- Co-authored-by: brighteyed <sergey.kondrikov@gmail.com>
40 lines
784 B
TypeScript
40 lines
784 B
TypeScript
export class SlideshowHistory {
|
|
private history: string[] = [];
|
|
private index = 0;
|
|
|
|
constructor(private onChange: (assetId: string) => void) {}
|
|
|
|
reset() {
|
|
this.history = [];
|
|
this.index = 0;
|
|
}
|
|
|
|
queue(assetId: string) {
|
|
this.history.push(assetId);
|
|
|
|
// If we were at the end of the slideshow history, move the index to the new end
|
|
if (this.index === this.history.length - 2) {
|
|
this.index++;
|
|
}
|
|
}
|
|
|
|
next(): boolean {
|
|
if (this.index === this.history.length - 1) {
|
|
return false;
|
|
}
|
|
|
|
this.index++;
|
|
this.onChange(this.history[this.index]);
|
|
return true;
|
|
}
|
|
|
|
previous(): boolean {
|
|
if (this.index === 0) {
|
|
return false;
|
|
}
|
|
|
|
this.index--;
|
|
this.onChange(this.history[this.index]);
|
|
return true;
|
|
}
|
|
}
|