mirror of
https://github.com/immich-app/immich.git
synced 2025-03-01 15:11:21 +01:00
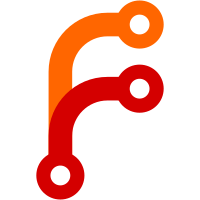
Now we only validate access token when we have one in the store and only send you to the login page when the response from the server is a 401 linter Co-authored-by: Alex Tran <alex.tran1502@gmail.com>
46 lines
1.7 KiB
Dart
46 lines
1.7 KiB
Dart
import 'dart:io';
|
|
|
|
import 'package:auto_route/auto_route.dart';
|
|
import 'package:immich_mobile/routing/router.dart';
|
|
import 'package:immich_mobile/shared/models/store.dart';
|
|
import 'package:immich_mobile/shared/services/api.service.dart';
|
|
import 'package:logging/logging.dart';
|
|
import 'package:openapi/api.dart';
|
|
|
|
class AuthGuard extends AutoRouteGuard {
|
|
final ApiService _apiService;
|
|
final _log = Logger("AuthGuard");
|
|
AuthGuard(this._apiService);
|
|
@override
|
|
void onNavigation(NavigationResolver resolver, StackRouter router) async {
|
|
resolver.next(true);
|
|
|
|
try {
|
|
// Look in the store for an access token
|
|
Store.get(StoreKey.accessToken);
|
|
|
|
// Validate the access token with the server
|
|
final res = await _apiService.authenticationApi.validateAccessToken();
|
|
if (res == null || res.authStatus != true) {
|
|
// If the access token is invalid, take user back to login
|
|
_log.fine('User token is invalid. Redirecting to login');
|
|
router.replaceAll([const LoginRoute()]);
|
|
}
|
|
} on StoreKeyNotFoundException catch (_) {
|
|
// If there is no access token, take us to the login page
|
|
_log.warning('No access token in the store.');
|
|
router.replaceAll([const LoginRoute()]);
|
|
return;
|
|
} on ApiException catch (e) {
|
|
// On an unauthorized request, take us to the login page
|
|
if (e.code == HttpStatus.unauthorized) {
|
|
_log.warning("Unauthorized access token.");
|
|
router.replaceAll([const LoginRoute()]);
|
|
return;
|
|
}
|
|
} catch (e) {
|
|
// Otherwise, this is not fatal, but we still log the warning
|
|
_log.warning('Error validating access token from server: $e');
|
|
}
|
|
}
|
|
}
|