mirror of
https://github.com/immich-app/immich.git
synced 2025-01-25 05:02:46 +01:00
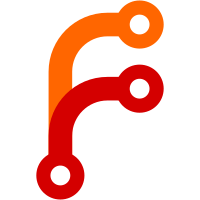
* custom `IsOptional` * added link to source * formatting * Update server/src/domain/domain.util.ts Co-authored-by: Jason Rasmussen <jrasm91@gmail.com> * nullable birth date endpoint * made `nullable` a property * formatting * removed unused dto * updated decorator arg * fixed album e2e tests * add null tests for auth e2e * add null test for person e2e * fixed tests * added null test for user e2e * removed unusued import * log key in test name * chore: add note about mobile not being able to use the endpoint --------- Co-authored-by: Jason Rasmussen <jrasm91@gmail.com>
98 lines
2 KiB
TypeScript
98 lines
2 KiB
TypeScript
import { AssetFaceEntity, PersonEntity } from '@app/infra/entities';
|
|
import { ApiProperty } from '@nestjs/swagger';
|
|
import { Transform, Type } from 'class-transformer';
|
|
import { IsArray, IsBoolean, IsDate, IsNotEmpty, IsString, ValidateNested } from 'class-validator';
|
|
import { Optional, toBoolean, ValidateUUID } from '../domain.util';
|
|
|
|
export class PersonUpdateDto {
|
|
/**
|
|
* Person name.
|
|
*/
|
|
@Optional()
|
|
@IsString()
|
|
name?: string;
|
|
|
|
/**
|
|
* Person date of birth.
|
|
* Note: the mobile app cannot currently set the birth date to null.
|
|
*/
|
|
@Optional({ nullable: true })
|
|
@IsDate()
|
|
@Type(() => Date)
|
|
@ApiProperty({ format: 'date' })
|
|
birthDate?: Date | null;
|
|
|
|
/**
|
|
* Asset is used to get the feature face thumbnail.
|
|
*/
|
|
@Optional()
|
|
@IsString()
|
|
featureFaceAssetId?: string;
|
|
|
|
/**
|
|
* Person visibility
|
|
*/
|
|
@Optional()
|
|
@IsBoolean()
|
|
isHidden?: boolean;
|
|
}
|
|
|
|
export class PeopleUpdateDto {
|
|
@IsArray()
|
|
@ValidateNested({ each: true })
|
|
@Type(() => PeopleUpdateItem)
|
|
people!: PeopleUpdateItem[];
|
|
}
|
|
|
|
export class PeopleUpdateItem extends PersonUpdateDto {
|
|
/**
|
|
* Person id.
|
|
*/
|
|
@IsString()
|
|
@IsNotEmpty()
|
|
id!: string;
|
|
}
|
|
|
|
export class MergePersonDto {
|
|
@ValidateUUID({ each: true })
|
|
ids!: string[];
|
|
}
|
|
|
|
export class PersonSearchDto {
|
|
@IsBoolean()
|
|
@Transform(toBoolean)
|
|
withHidden?: boolean = false;
|
|
}
|
|
|
|
export class PersonResponseDto {
|
|
id!: string;
|
|
name!: string;
|
|
@ApiProperty({ format: 'date' })
|
|
birthDate!: Date | null;
|
|
thumbnailPath!: string;
|
|
isHidden!: boolean;
|
|
}
|
|
|
|
export class PeopleResponseDto {
|
|
@ApiProperty({ type: 'integer' })
|
|
total!: number;
|
|
|
|
@ApiProperty({ type: 'integer' })
|
|
visible!: number;
|
|
|
|
people!: PersonResponseDto[];
|
|
}
|
|
|
|
export function mapPerson(person: PersonEntity): PersonResponseDto {
|
|
return {
|
|
id: person.id,
|
|
name: person.name,
|
|
birthDate: person.birthDate,
|
|
thumbnailPath: person.thumbnailPath,
|
|
isHidden: person.isHidden,
|
|
};
|
|
}
|
|
|
|
export function mapFace(face: AssetFaceEntity): PersonResponseDto {
|
|
return mapPerson(face.person);
|
|
}
|