mirror of
https://github.com/immich-app/immich.git
synced 2025-01-10 13:56:47 +01:00
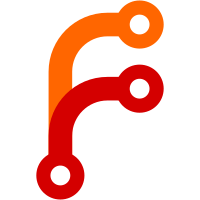
* refactor: auth * chore: tests * Remove await on non-async method * refactor: constants * chore: remove extra async Co-authored-by: Alex Tran <alex.tran1502@gmail.com>
44 lines
1.3 KiB
TypeScript
44 lines
1.3 KiB
TypeScript
import { plainToInstance } from 'class-transformer';
|
|
import { validateSync } from 'class-validator';
|
|
import { SignUpDto } from './sign-up.dto';
|
|
|
|
describe('SignUpDto', () => {
|
|
it('should require all fields', () => {
|
|
const dto = plainToInstance(SignUpDto, {
|
|
email: '',
|
|
password: '',
|
|
firstName: '',
|
|
lastName: '',
|
|
});
|
|
const errors = validateSync(dto);
|
|
expect(errors).toHaveLength(4);
|
|
expect(errors[0].property).toEqual('email');
|
|
expect(errors[1].property).toEqual('password');
|
|
expect(errors[2].property).toEqual('firstName');
|
|
expect(errors[3].property).toEqual('lastName');
|
|
});
|
|
|
|
it('should require a valid email', () => {
|
|
const dto = plainToInstance(SignUpDto, {
|
|
email: 'immich.com',
|
|
password: 'password',
|
|
firstName: 'first name',
|
|
lastName: 'last name',
|
|
});
|
|
const errors = validateSync(dto);
|
|
expect(errors).toHaveLength(1);
|
|
expect(errors[0].property).toEqual('email');
|
|
});
|
|
|
|
it('should make the email all lowercase', () => {
|
|
const dto = plainToInstance(SignUpDto, {
|
|
email: 'TeSt@ImMiCh.com',
|
|
password: 'password',
|
|
firstName: 'first name',
|
|
lastName: 'last name',
|
|
});
|
|
const errors = validateSync(dto);
|
|
expect(errors).toHaveLength(0);
|
|
expect(dto.email).toEqual('test@immich.com');
|
|
});
|
|
});
|