mirror of
https://github.com/immich-app/immich.git
synced 2025-04-14 20:16:24 +02:00
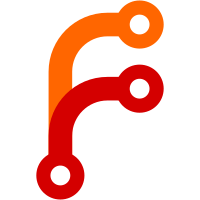
* fix(web): previous/next asset navigation * Apply suggestions from code review Co-authored-by: Thomas <9749173+uhthomas@users.noreply.github.com> * Call setViewingAsset once * Make code more readable * Avoid recursive call * Simplify return statement * Set position of the bucket to Unknown --------- Co-authored-by: Thomas <9749173+uhthomas@users.noreply.github.com> Co-authored-by: Alex Tran <alex.tran1502@gmail.com>
58 lines
1.2 KiB
TypeScript
58 lines
1.2 KiB
TypeScript
import type { AssetResponseDto } from '@api';
|
|
|
|
export enum BucketPosition {
|
|
Above = 'above',
|
|
Below = 'below',
|
|
Visible = 'visible',
|
|
Unknown = 'unknown',
|
|
}
|
|
|
|
export class AssetBucket {
|
|
/**
|
|
* The DOM height of the bucket in pixel
|
|
* This value is first estimated by the number of asset and later is corrected as the user scroll
|
|
*/
|
|
bucketHeight!: number;
|
|
bucketDate!: string;
|
|
assets!: AssetResponseDto[];
|
|
cancelToken!: AbortController;
|
|
position!: BucketPosition;
|
|
}
|
|
|
|
export class AssetGridState {
|
|
/**
|
|
* The total height of the timeline in pixel
|
|
* This value is first estimated by the number of asset and later is corrected as the user scroll
|
|
*/
|
|
timelineHeight = 0;
|
|
|
|
/**
|
|
* The fixed viewport height in pixel
|
|
*/
|
|
viewportHeight = 0;
|
|
|
|
/**
|
|
* The fixed viewport width in pixel
|
|
*/
|
|
viewportWidth = 0;
|
|
|
|
/**
|
|
* List of bucket information
|
|
*/
|
|
buckets: AssetBucket[] = [];
|
|
|
|
/**
|
|
* Total assets that have been loaded
|
|
*/
|
|
assets: AssetResponseDto[] = [];
|
|
|
|
/**
|
|
* Total assets that have been loaded along with additional data
|
|
*/
|
|
loadedAssets: Record<string, number> = {};
|
|
|
|
/**
|
|
* User that owns assets
|
|
*/
|
|
userId: string | undefined;
|
|
}
|