mirror of
https://github.com/immich-app/immich.git
synced 2025-01-10 05:46:46 +01:00
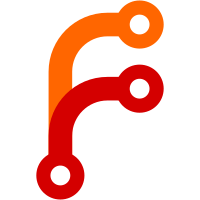
* Add new cli * Remove old readme * Add documentation to readme file * Add github workflow tests for cli * Fix typo in docs * Add usage info to readme * Add package-lock.json * Fix tsconfig * Cleanup * Fix lint * Cleanup package.json * Fix accidental server change * Remove rootdir from cli * Remove tsbuildinfo * Add prettierignore * Make CLI use internal openapi specs * Add ignore and dry-run features * Sort paths alphabetically * Don't remove substring * Remove shorthand for delete * Remove unused import * Remove chokidar * Set correct openapi cli generator script * Add progress bar * Rename target to asset * Add deletion progress bar * Ignore require statement * Use read streams instead of readfile * Fix github feedback * Fix upload requires * More github comments * Cleanup messages * Cleaner pattern concats * Github comments --------- Co-authored-by: Alex Tran <alex.tran1502@gmail.com>
50 lines
1.4 KiB
TypeScript
50 lines
1.4 KiB
TypeScript
import {
|
|
AlbumApi,
|
|
APIKeyApi,
|
|
AssetApi,
|
|
AuthenticationApi,
|
|
Configuration,
|
|
JobApi,
|
|
OAuthApi,
|
|
ServerInfoApi,
|
|
SystemConfigApi,
|
|
UserApi,
|
|
} from './open-api';
|
|
import { ApiConfiguration } from '../cores/api-configuration';
|
|
|
|
export class ImmichApi {
|
|
public userApi: UserApi;
|
|
public albumApi: AlbumApi;
|
|
public assetApi: AssetApi;
|
|
public authenticationApi: AuthenticationApi;
|
|
public oauthApi: OAuthApi;
|
|
public serverInfoApi: ServerInfoApi;
|
|
public jobApi: JobApi;
|
|
public keyApi: APIKeyApi;
|
|
public systemConfigApi: SystemConfigApi;
|
|
|
|
private readonly config;
|
|
public readonly apiConfiguration: ApiConfiguration;
|
|
|
|
constructor(instanceUrl: string, apiKey: string) {
|
|
this.apiConfiguration = new ApiConfiguration(instanceUrl, apiKey);
|
|
this.config = new Configuration({
|
|
basePath: instanceUrl,
|
|
baseOptions: {
|
|
headers: {
|
|
'x-api-key': apiKey,
|
|
},
|
|
},
|
|
});
|
|
|
|
this.userApi = new UserApi(this.config);
|
|
this.albumApi = new AlbumApi(this.config);
|
|
this.assetApi = new AssetApi(this.config);
|
|
this.authenticationApi = new AuthenticationApi(this.config);
|
|
this.oauthApi = new OAuthApi(this.config);
|
|
this.serverInfoApi = new ServerInfoApi(this.config);
|
|
this.jobApi = new JobApi(this.config);
|
|
this.keyApi = new APIKeyApi(this.config);
|
|
this.systemConfigApi = new SystemConfigApi(this.config);
|
|
}
|
|
}
|