mirror of
https://github.com/immich-app/immich.git
synced 2025-01-09 21:36:46 +01:00
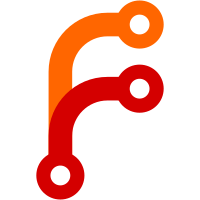
* refactor(server): tsconfigs * chore: dummy commit * fix: start.sh * chore: restore original entry scripts
46 lines
1.4 KiB
TypeScript
46 lines
1.4 KiB
TypeScript
import { newStorageRepositoryMock } from '@test';
|
|
import { IStorageRepository } from '.';
|
|
import { StorageService } from './storage.service';
|
|
|
|
describe(StorageService.name, () => {
|
|
let sut: StorageService;
|
|
let storageMock: jest.Mocked<IStorageRepository>;
|
|
|
|
beforeEach(async () => {
|
|
storageMock = newStorageRepositoryMock();
|
|
sut = new StorageService(storageMock);
|
|
});
|
|
|
|
it('should work', () => {
|
|
expect(sut).toBeDefined();
|
|
});
|
|
|
|
describe('init', () => {
|
|
it('should create the library folder on initialization', () => {
|
|
sut.init();
|
|
expect(storageMock.mkdirSync).toHaveBeenCalledWith('upload/library');
|
|
});
|
|
});
|
|
|
|
describe('handleDeleteFiles', () => {
|
|
it('should handle null values', async () => {
|
|
await sut.handleDeleteFiles({ files: [undefined, null] });
|
|
|
|
expect(storageMock.unlink).not.toHaveBeenCalled();
|
|
});
|
|
|
|
it('should handle an error removing a file', async () => {
|
|
storageMock.unlink.mockRejectedValue(new Error('something-went-wrong'));
|
|
|
|
await sut.handleDeleteFiles({ files: ['path/to/something'] });
|
|
|
|
expect(storageMock.unlink).toHaveBeenCalledWith('path/to/something');
|
|
});
|
|
|
|
it('should remove the file', async () => {
|
|
await sut.handleDeleteFiles({ files: ['path/to/something'] });
|
|
|
|
expect(storageMock.unlink).toHaveBeenCalledWith('path/to/something');
|
|
});
|
|
});
|
|
});
|