mirror of
https://github.com/immich-app/immich.git
synced 2025-01-10 13:56:47 +01:00
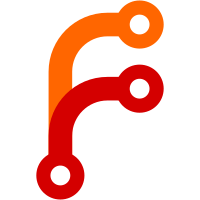
* feat: explore * chore: generate open api * styling explore page * styling no result page * style overlay * style: bluring text on thumbnail card for readability * explore page tweaks * fix(web): search urls * feat(web): use objects for things * feat(server): filter by motion, sort by createdAt * More styling * better navigation --------- Co-authored-by: Alex Tran <alex.tran1502@gmail.com> Co-authored-by: Michel Heusschen <59014050+michelheusschen@users.noreply.github.com>
61 lines
2 KiB
TypeScript
61 lines
2 KiB
TypeScript
import { AssetEntity, AssetType } from '@app/infra/db/entities';
|
|
import { assetEntityStub, newAssetRepositoryMock, newJobRepositoryMock } from '../../test';
|
|
import { AssetService, IAssetRepository } from '../asset';
|
|
import { IJobRepository, JobName } from '../job';
|
|
|
|
describe(AssetService.name, () => {
|
|
let sut: AssetService;
|
|
let assetMock: jest.Mocked<IAssetRepository>;
|
|
let jobMock: jest.Mocked<IJobRepository>;
|
|
|
|
it('should work', () => {
|
|
expect(sut).toBeDefined();
|
|
});
|
|
|
|
beforeEach(async () => {
|
|
assetMock = newAssetRepositoryMock();
|
|
jobMock = newJobRepositoryMock();
|
|
sut = new AssetService(assetMock, jobMock);
|
|
});
|
|
|
|
describe(`handle asset upload`, () => {
|
|
it('should process an uploaded video', async () => {
|
|
const data = { asset: { type: AssetType.VIDEO } as AssetEntity, fileName: 'video.mp4' };
|
|
|
|
await expect(sut.handleAssetUpload(data)).resolves.toBeUndefined();
|
|
|
|
expect(jobMock.queue).toHaveBeenCalledTimes(3);
|
|
expect(jobMock.queue.mock.calls).toEqual([
|
|
[{ name: JobName.GENERATE_JPEG_THUMBNAIL, data }],
|
|
[{ name: JobName.VIDEO_CONVERSION, data }],
|
|
[{ name: JobName.EXTRACT_VIDEO_METADATA, data }],
|
|
]);
|
|
});
|
|
|
|
it('should process an uploaded image', async () => {
|
|
const data = { asset: { type: AssetType.IMAGE } as AssetEntity, fileName: 'image.jpg' };
|
|
|
|
await sut.handleAssetUpload(data);
|
|
|
|
expect(jobMock.queue).toHaveBeenCalledTimes(2);
|
|
expect(jobMock.queue.mock.calls).toEqual([
|
|
[{ name: JobName.GENERATE_JPEG_THUMBNAIL, data }],
|
|
[{ name: JobName.EXIF_EXTRACTION, data }],
|
|
]);
|
|
});
|
|
});
|
|
|
|
describe('save', () => {
|
|
it('should save an asset', async () => {
|
|
assetMock.save.mockResolvedValue(assetEntityStub.image);
|
|
|
|
await sut.save(assetEntityStub.image);
|
|
|
|
expect(assetMock.save).toHaveBeenCalledWith(assetEntityStub.image);
|
|
expect(jobMock.queue).toHaveBeenCalledWith({
|
|
name: JobName.SEARCH_INDEX_ASSET,
|
|
data: { asset: assetEntityStub.image },
|
|
});
|
|
});
|
|
});
|
|
});
|