mirror of
https://github.com/immich-app/immich.git
synced 2024-12-29 15:11:58 +00:00
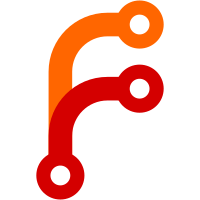
* feat(mobile): album view sort order * feat: add error message * refactor(mobile): album page (#14659) * refactor album page * update lint rule * const record * fix: updating sort order when pull to refresh --------- Co-authored-by: Alex <alex.tran1502@gmail.com> * Move sort toggle button to bottom sheet menu * chore: revert multiselectgrid loading status * chore: revert multiselectgrid loading status --------- Co-authored-by: Mert <101130780+mertalev@users.noreply.github.com>
56 lines
1.7 KiB
Dart
56 lines
1.7 KiB
Dart
import 'package:auto_route/auto_route.dart';
|
|
import 'package:flutter/material.dart';
|
|
import 'package:flutter_hooks/flutter_hooks.dart';
|
|
import 'package:hooks_riverpod/hooks_riverpod.dart';
|
|
import 'package:immich_mobile/entities/user.entity.dart';
|
|
import 'package:immich_mobile/providers/album/current_album.provider.dart';
|
|
import 'package:immich_mobile/routing/router.dart';
|
|
import 'package:immich_mobile/widgets/common/user_circle_avatar.dart';
|
|
|
|
class AlbumSharedUserIcons extends HookConsumerWidget {
|
|
const AlbumSharedUserIcons({super.key});
|
|
|
|
@override
|
|
Widget build(BuildContext context, WidgetRef ref) {
|
|
final sharedUsers = useRef<List<User>>(const []);
|
|
sharedUsers.value = ref.watch(
|
|
currentAlbumProvider.select((album) {
|
|
if (album == null) {
|
|
return const [];
|
|
}
|
|
|
|
if (album.sharedUsers.length == sharedUsers.value.length) {
|
|
return sharedUsers.value;
|
|
}
|
|
|
|
return album.sharedUsers.toList(growable: false);
|
|
}),
|
|
);
|
|
|
|
if (sharedUsers.value.isEmpty) {
|
|
return const SizedBox();
|
|
}
|
|
|
|
return GestureDetector(
|
|
onTap: () => context.pushRoute(AlbumOptionsRoute()),
|
|
child: SizedBox(
|
|
height: 50,
|
|
child: ListView.builder(
|
|
padding: const EdgeInsets.only(left: 16),
|
|
scrollDirection: Axis.horizontal,
|
|
itemBuilder: ((context, index) {
|
|
return Padding(
|
|
padding: const EdgeInsets.only(right: 8.0),
|
|
child: UserCircleAvatar(
|
|
user: sharedUsers.value[index],
|
|
radius: 18,
|
|
size: 36,
|
|
),
|
|
);
|
|
}),
|
|
itemCount: sharedUsers.value.length,
|
|
),
|
|
),
|
|
);
|
|
}
|
|
}
|