mirror of
https://github.com/immich-app/immich.git
synced 2024-12-29 15:11:58 +00:00
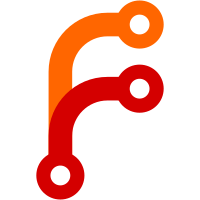
* curating assets with albums to upload * sorting for background backup * background upload works * transform fields string array to javascript array * send json array * generate sql * refactor upload callback * remove albums info from upload payload * mechanism to create album on album selection * album creation * Sync to upload album * Remove unused service * unify name changes * Add mechanism to sync uploaded assets to albums * Put add to album operation after updating the UI state * clean up * background album sync * add to album in background context * remove add to album in callback * refactor * refactor * refactor * fix: make sure all selected albums are selected for building upload candidate * clean up * add manual sync button * lint * revert server changes * pr feedback * revert time filtering * const * sync album on manual upload * linting * pr feedback and proper time filtering * wording
42 lines
1.1 KiB
Dart
42 lines
1.1 KiB
Dart
import 'package:immich_mobile/models/backup/backup_candidate.model.dart';
|
|
|
|
class SuccessUploadAsset {
|
|
final BackupCandidate candidate;
|
|
final String remoteAssetId;
|
|
final bool isDuplicate;
|
|
|
|
SuccessUploadAsset({
|
|
required this.candidate,
|
|
required this.remoteAssetId,
|
|
required this.isDuplicate,
|
|
});
|
|
|
|
SuccessUploadAsset copyWith({
|
|
BackupCandidate? candidate,
|
|
String? remoteAssetId,
|
|
bool? isDuplicate,
|
|
}) {
|
|
return SuccessUploadAsset(
|
|
candidate: candidate ?? this.candidate,
|
|
remoteAssetId: remoteAssetId ?? this.remoteAssetId,
|
|
isDuplicate: isDuplicate ?? this.isDuplicate,
|
|
);
|
|
}
|
|
|
|
@override
|
|
String toString() =>
|
|
'SuccessUploadAsset(asset: $candidate, remoteAssetId: $remoteAssetId, isDuplicate: $isDuplicate)';
|
|
|
|
@override
|
|
bool operator ==(covariant SuccessUploadAsset other) {
|
|
if (identical(this, other)) return true;
|
|
|
|
return other.candidate == candidate &&
|
|
other.remoteAssetId == remoteAssetId &&
|
|
other.isDuplicate == isDuplicate;
|
|
}
|
|
|
|
@override
|
|
int get hashCode =>
|
|
candidate.hashCode ^ remoteAssetId.hashCode ^ isDuplicate.hashCode;
|
|
}
|