mirror of
https://github.com/immich-app/immich.git
synced 2025-01-04 10:56:47 +01:00
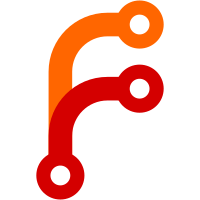
* First version of video looping for the web * Use prop for slideshow state * refactor asset settings and add autoloop video setting * rename variables and adjust description * loop videos based on user settings in gallery viewer * make asset viewer setting a stateless widget * do not update video playback value if looping is enabled * add some translations * adjust description * add missing id * WIP * chore: clean up --------- Co-authored-by: Alex <alex.tran1502@gmail.com> Co-authored-by: Jason Rasmussen <jrasm91@gmail.com>
48 lines
1.4 KiB
Dart
48 lines
1.4 KiB
Dart
import 'package:chewie/chewie.dart';
|
|
import 'package:flutter/material.dart';
|
|
import 'package:hooks_riverpod/hooks_riverpod.dart';
|
|
import 'package:immich_mobile/utils/hooks/chewiew_controller_hook.dart';
|
|
import 'package:immich_mobile/widgets/asset_viewer/custom_video_player_controls.dart';
|
|
import 'package:video_player/video_player.dart';
|
|
|
|
class VideoPlayerViewer extends HookConsumerWidget {
|
|
final VideoPlayerController controller;
|
|
final bool isMotionVideo;
|
|
final Widget? placeholder;
|
|
final Duration hideControlsTimer;
|
|
final bool showControls;
|
|
final bool showDownloadingIndicator;
|
|
final bool loopVideo;
|
|
|
|
const VideoPlayerViewer({
|
|
super.key,
|
|
required this.controller,
|
|
required this.isMotionVideo,
|
|
this.placeholder,
|
|
required this.hideControlsTimer,
|
|
required this.showControls,
|
|
required this.showDownloadingIndicator,
|
|
required this.loopVideo,
|
|
});
|
|
|
|
@override
|
|
Widget build(BuildContext context, WidgetRef ref) {
|
|
final chewie = useChewieController(
|
|
controller: controller,
|
|
controlsSafeAreaMinimum: const EdgeInsets.only(
|
|
bottom: 100,
|
|
),
|
|
placeholder: SizedBox.expand(child: placeholder),
|
|
customControls: CustomVideoPlayerControls(
|
|
hideTimerDuration: hideControlsTimer,
|
|
),
|
|
showControls: showControls && !isMotionVideo,
|
|
hideControlsTimer: hideControlsTimer,
|
|
loopVideo: loopVideo,
|
|
);
|
|
|
|
return Chewie(
|
|
controller: chewie,
|
|
);
|
|
}
|
|
}
|