mirror of
https://github.com/ohmyzsh/ohmyzsh.git
synced 2024-11-12 08:50:08 +00:00
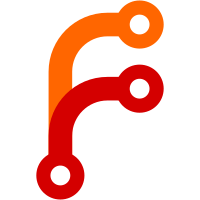
Previously, OS detection would happen on each invocation. This makes it happen once (unless it fails, in which case it will try again on the next invocation). This has the additional benefit of localizing the platform-specific checks and commands, too, versus spreading them out in separate functions.
74 lines
2.4 KiB
Bash
74 lines
2.4 KiB
Bash
# System clipboard integration
|
|
#
|
|
# This file has support for doing system clipboard copy and paste operations
|
|
# from the command line in a generic cross-platform fashion.
|
|
#
|
|
# On OS X and Windows, the main system clipboard or "pasteboard" is used. On other
|
|
# Unix-like OSes, this considers the X Windows CLIPBOARD selection to be the
|
|
# "system clipboard", and the X Windows `xclip` command must be installed.
|
|
|
|
# clipcopy - Copy data to clipboard
|
|
#
|
|
# Usage:
|
|
#
|
|
# <command> | clipcopy - copies stdin to clipboard
|
|
#
|
|
# clipcopy <file> - copies a file's contents to clipboard
|
|
#
|
|
#
|
|
##
|
|
#
|
|
# clippaste - "Paste" data from clipboard to stdout
|
|
#
|
|
# Usage:
|
|
#
|
|
# clippaste - writes clipboard's contents to stdout
|
|
#
|
|
# clippaste | <command> - pastes contents and pipes it to another process
|
|
#
|
|
# clippaste > <file> - paste contents to a file
|
|
#
|
|
# Examples:
|
|
#
|
|
# # Pipe to another process
|
|
# clippaste | grep foo
|
|
#
|
|
# # Paste to a file
|
|
# clippaste > file.txt
|
|
#
|
|
function detect-clipboard() {
|
|
emulate -L zsh
|
|
|
|
if [[ $OSTYPE == darwin* ]]; then
|
|
function clipcopy() { pbcopy < "${1:-/dev/stdin}"; }
|
|
function clippaste() { pbpaste; }
|
|
elif [[ $OSTYPE == cygwin* ]]; then
|
|
function clipcopy() { cat "${1:-/dev/stdin}" > /dev/clipboard; }
|
|
function clippaste() { cat /dev/clipboard; }
|
|
elif (( $+commands[xclip] )); then
|
|
function clipcopy() { xclip -in -selection clipboard < "${1:-/dev/stdin}"; }
|
|
function clippaste() { xclip -out -selection clipboard; }
|
|
elif (( $+commands[xsel] )); then
|
|
function clipcopy() { xsel --clipboard --input < "${1:-/dev/stdin}"; }
|
|
function clippaste() { xsel --clipboard --output; }
|
|
else
|
|
function _retry_clipboard_detection_or_fail() {
|
|
local clipcmd="${1}"; shift
|
|
if detect-clipboard; then
|
|
"${clipcmd}" "$@"
|
|
else
|
|
print "${clipcmd}: Platform $OSTYPE not supported or xclip/xsel not installed" >&2
|
|
return 1
|
|
fi
|
|
}
|
|
function clipcopy() { _retry_clipboard_detection_or_fail clipcopy "$@"; }
|
|
function cilppaste() { _retry_clipboard_detection_or_fail clippaste "$@"; }
|
|
return 1
|
|
fi
|
|
}
|
|
|
|
# Detect at startup. A non-zero exit here indicates that the dummy clipboards were set,
|
|
# which is not really an error. If the user calls them, they will attempt to redetect
|
|
# (for example, perhaps the user has now installed xclip) and then either print an error
|
|
# or proceed successfully.
|
|
detect-clipboard || true
|